|
Design Pattern |
|
I. Observer Design Pattern
This design pattern defines one to many
dependency between the objects so that if an
object changes its state then all the dependent
objects are notified and updated automatically.
It is mainly used, to maintain consistency
between objects, support to broadcast
communication, to maintain classes for further
use by making them loosely coupled, to make
GUI application and many more. Java API
provides a built-in Observable class and
Observer interface.
Here we are taking an example just to
demonstrate that how observer pattern works,
for this, the example creates two windows. The
first window takes the input from the user and
the second window displays this input. As soon
as the data is entered in the textfield and enter
button is pressed, the second window gets the
message and displays it with a dialog. The
example uses the private inner class.
import javax.swing.*;
import java.awt.event.*;
import java.util.*;
class ShowForm extends JFrame {
InputFormObserverinputformobserver= newInputFormObserver();
InputForm inputForm ;
Observable obsInput;
JTextField display;
//...
public ShowForm() {
addWindowListener(new WindowAdapter() {
public void windowClosing(WindowEvent e) {
System.exit(0);
}});
inputForm = new InputForm();
obsInput = inputForm.getInputInfo();
obsInput.addObserver(inputformobserver);
display = new JTextField(10);
display.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
}
});
getContentPane().add(display);
setTitle("Observer form");
setSize(200,100);
setLocation(200,100);
setVisible(true); |
|
}
private class InputFormObserver implements Observer {
public void update(Observable ob, Object o) {
doSomeUpdate();
if (obsInput.countObservers()>0)
obsInput.deleteObservers();
obsInput = inputForm.getInputInfo();
obsInput.addObserver(inputformobserver);
}
}
public void doSomeUpdate() {
display.setText(inputForm.getText());
JOptionPane.showMessageDialog(ShowForm.this,
"This form has been updated");
}
public static void main(String args[]) {
ShowForm df = new ShowForm();
}
}
class InputForm extends JFrame {
public InformDisplay inform = new InformDisplay();
//...
JTextField input= new JTextField(10);
public InputForm() {
JPanel panel= new JPanel();
input.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
inform.notifyObservers();
}
});
panel.add(new JLabel("Enter: "));
panel.add(input);
addWindowListener(new WindowAdapter() {
public void windowClosing(WindowEvent e) {
System.exit(0);
}});
getContentPane().add(panel);
setTitle("Observable form");
setSize(200,100);
setVisible(true);
}
public Observable getInputInfo() {
return inform;
}
public String getText() {
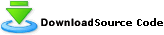
|
|
Feb
2008 | Java Jazz Up | 59 |
|
|
View All Topics |
All Pages of this Issue |
Pages:
1,
2,
3,
4,
5,
6,
7,
8,
9,
10,
11,
12,
13,
14,
15,
16,
17,
18,
19,
20,
21,
22,
23,
24,
25,
26,
27,
28,
29,
30,
31,
32,
33,
34,
35,
36,
37,
38,
39,
40,
41,
42,
43,
44,
45,
46,
47,
48,
49,
50,
51,
52,
53 ,
54,
55,
56,
57,
58,
59,
60,
61,
62,
63 ,
64,
65 ,
66 ,
67 ,
68 ,
69 ,
70 ,
71 ,
72 ,
Download PDF |
|
|
|
|
|
|
|
|
|