|
Design Pattern |
|
Doctor.java
class Doctor implements Person {
private double feeperPatient;
private int NoOfPatient;
private String name;
public Doctor(String name, double f, int n) {
this.name = name;
setFeeperPatient(f);
setNoOfPatient(n);
}
void setFeeperPatient(double f) {
if (f > 0)
feeperPatient = f;
else
feeperPatient = 0;
}
void setNoOfPatient(int n) {
if ( n > 0)
NoOfPatient = n;
else
NoOfPatient = 0;
}
public String getName() {
return name;
}
public double income() {
return NoOfPatient * feeperPatient;
}
public String toString() {
return “Doctor : “ + getName();
}}
PersonTest.java
import java.util.Iterator;
import java.util.ArrayList;
import java.util.List;
class PersonTest {
public static void main(String[] args) {
List list = new ArrayList();
list.add(new Teacher(“Bill”, 800.00));
list.add(new Doctor(“Al”, 2.5, 200));
list.add(new Teacher(“Peter”, 1200.00));
list.add(new Doctor(“Mark”, 4.5, 333)); |
|
System.out.println(“Use built-in iterator:”);
Iterator iterator = list.iterator();
while(iterator.hasNext()) {
Person pr = (Person)iterator.next();
if (pr instanceof Teacher) {
System.out.print(“Teacher has “ + pr + “
income $”);
System.out.println(pr.income());
}}}}
The above example also shows a dynamic binding feature, which is popular in Object-Oriented realm.If you want to pick up a specific object from
the aggregated list, you may use the following code.
II. Mediator Pattern
Mediator pattern takes an object that puts the logic to manages state changes of other objects rather than distributing the logic among the
various objects that results in decreasing the coupling between the other objects. Define an object that encapsulates how a set of objects interacts. Mediator promotes loose coupling by keeping objects from referring to each other explicitly, and lets you vary their interaction independently. Lets try to under stand more clearly, When we starts developing an application that have few classes and these classes interact with each other just to produce a result. As soon as the application becomes large then the logic becomes more complex and functionality increases. In such condition it is difficult to maintain this code then Mediator pattern solves the problem by maintaining the code. It loosecouples to the classes so that only one class (Mediator) has the information about rest of the classes, rest of the classes only interacts
with the Mediator.
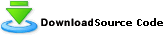
|
|
Jan
2007 | Java Jazz Up | 91 |
|
|
View All Topics |
All Pages of this Issue |
Pages:
1,
2,
3,
4,
5,
6,
7,
8,
9,
10,
11,
12,
13,
14,
15,
16,
17,
18,
19,
20,
21,
22,
23,
24,
25,
26,
27,
28,
29,
30,
31,
32,
33,
34,
35,
36,
37,
38,
39,
40,
41,
42,
43,
44,
45,
46,
47,
48,
49,
50,
51,
52,
53 ,
54,
55,
56,
57,
58,
59,
60,
61,
62,
63 ,
64,
65 ,
66 ,
67 ,
68 ,
69 ,
70 ,
71 ,
72 ,
73 ,
74 ,
75 ,
76 ,
77 ,
78 ,
79 ,
80 ,
81 ,
82 ,
83,
84 ,
85 ,
86,
87 ,
88,
89 ,
90 ,
91 ,
92 ,
93 ,
94 ,
95 ,
96 ,
97 ,
98 ,
99 ,
100 ,
101 ,
102 ,
103,
104 ,
105 ,
106,
107,
Download PDF |
|
|
|
|
|
|
|
|
|