|
Hibernate with Annotation |
|
@Table(name = “employee”)
public class Employee implements
Serializable {
public Employee() {
}
@Id
@Column(name = “id”)
Integer id;
@Column(name = “name”)
String name;
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
In the EJB word the entities represent the
persistence object. This can be achieved by
@Entity at the class level. @Table(name = “employee”) annotation tells the entity is
mapped with the table employee in the
database. Mapped classes must declare the
primary key column of the database table. Most
classes will also have a Java-Beans-style
property holding the unique identifier of an
instance. The @Id element defines the mapping
from that property to the primary key column.
@Column is used to map the entities with the
column in the database.
Step 4: Real application file which has the
actual logic manipulate the POJO
The following code demonstrates how to store
an entity in the database. The code is identical
as you have used in the Hibernate applications.
|
|
package net.roseindia;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.hibernate.Transaction;
public class Example1 {
/**
* @param args
*/
public static void main(String[] args)
throws Exception {
/** Getting the Session Factory and
session */
SessionFactory session =
HibernateUtil.getSessionFactory();
Session sess =
session.getCurrentSession();
/** Starting the Transaction */
Transaction tx = sess.beginTransaction();
/** Creating POJO */
Employee pojo = new Employee();
pojo.setId(new Integer(5));
pojo.setName(“XYZ”);
/** Saving POJO */
sess.save(pojo);
/** Commiting the changes */
tx.commit();
System.out.println(“Record Inserted”);
/** Closing Session */
session.close();
}
}
Output:
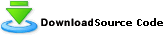
|
|
|
Jan 2008 | Java Jazz Up |38 |
|
|
|
View All Topics |
All Pages of this Issue |
Pages:
1,
2,
3,
4,
5,
6,
7,
8,
9,
10,
11,
12,
13,
14,
15,
16,
17,
18,
19,
20,
21,
22,
23,
24,
25,
26,
27,
28,
29,
30,
31,
32,
33,
34,
35,
36,
37,
38,
39,
40,
41,
42,
43,
44,
45,
46,
47,
48,
49,
50,
51,
52,
53 ,
54,
55,
56,
57,
58,
59,
60,
61,
62,
63 ,
64,
65 ,
66 ,
67 ,
68 ,
69 ,
70 ,
71 ,
72 ,
73 ,
74 ,
75 ,
76 ,
77 ,
78 ,
79 ,
80 ,
81 ,
82 ,
83,
84 ,
85 ,
86,
87 ,
88,
89 ,
90 ,
91 ,
92 ,
93 ,
94 ,
95 ,
96 ,
97 ,
98 ,
99 ,
100 ,
101 ,
102 ,
103,
104 ,
105 ,
106,
107,
Download PDF |
|
|
|
|
|
|