|
Design Pattern |
|
Behavioral Patterns Behavioral patterns are
those patterns, which are specifically concerned
with communication (interaction) between the
objects. The interactions between the objects
should be such that they are talking to each
other and are still loosely coupled. The loose
coupling is the key to n-tier architectures. In
this, the implementations and the client should
be loosely coupled in order to avoid hard coding
and dependencies. The behavioral patterns are:
1. Chain of Responsibility Pattern
2. Command Pattern
3. Interpreter Pattern
4. Iterator Pattern
5. Mediator Pattern
6. Momento Pattern
7. Observer Pattern
8. State Pattern
9. Strategy Pattern
10. Template Pattern
11. Visitor Pattern
In this Issue, we are going to discuss only the Iterator, Mediator, and the Memento design patterns.
Iterator Pattern
Iterator pattern is the mechanism of accessing all the objects in a collection. To sequentially access the objects of a collection, the iterator pattern defines an interface containing the methods that access the objects of a collection or the aggregate objects without having knowledge about its internal representation. It provides uniform interface to traverse collections of all kinds. Aggregate objects are the objects containing the group of objects as a unit. We can also referred them as container or collection. Hash table and linked list are the examples of aggregate objects.
Example: Lets take an example of employees of a software company and their section, then we add some enumeration capabilities to the Employee class. This class is the collection of employees having their names; employee id and their department and these employees arestored in a Vector. |
|
iNow we simply the enumeration of the Vector itself just to obtain the enumeration of all the employees of the collection. Filtered Enumeration: Suppose we want the employees of the development section. This requires a special enumeration class that access the employees belong only to the development department. The element() method we have defined provides filtered access. Now Enumeration that only returns employees related to the development section is required.
Person.java
import java.util.*;
interface Person {
public abstract double income();
}
Teacher.java
class Teacher implements Person {
private double MonthlyIncome;
private String name;
public Teacher(String name, double i) {
this.name = name;
setMonthlyIncome(i);
}
void setMonthlyIncome(double i) {
if (i > 0) {
MonthlyIncome = i;
} else
MonthlyIncome = 0;
}
public double income() {
return MonthlyIncome;
}
public String getName() {
return name;
}
public String toString() {
return “Teacher: “ + getName();
}}
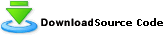
|
|
Jan
2008 | Java Jazz Up | 90 |
|
|
View All Topics |
All Pages of this Issue |
Pages:
1,
2,
3,
4,
5,
6,
7,
8,
9,
10,
11,
12,
13,
14,
15,
16,
17,
18,
19,
20,
21,
22,
23,
24,
25,
26,
27,
28,
29,
30,
31,
32,
33,
34,
35,
36,
37,
38,
39,
40,
41,
42,
43,
44,
45,
46,
47,
48,
49,
50,
51,
52,
53 ,
54,
55,
56,
57,
58,
59,
60,
61,
62,
63 ,
64,
65 ,
66 ,
67 ,
68 ,
69 ,
70 ,
71 ,
72 ,
73 ,
74 ,
75 ,
76 ,
77 ,
78 ,
79 ,
80 ,
81 ,
82 ,
83,
84 ,
85 ,
86,
87 ,
88,
89 ,
90 ,
91 ,
92 ,
93 ,
94 ,
95 ,
96 ,
97 ,
98 ,
99 ,
100 ,
101 ,
102 ,
103,
104 ,
105 ,
106,
107,
Download PDF |
|
|
|
|
|
|
|
|
|